Developing custom app
Requirements
- Custom widget in development mode
- Widget slug
- Custom widget example "Accordion widget" https://github.com/happeo/accordion-widget
- Edit permissions to a page
Developing in Pages
Let's start by adding your new custom widget into a page. To do that open a new page and from the widget selector select your new widget. The widget has a tag after it that says "In development". Note that you may need to scroll to it if there are a lot of widgets.
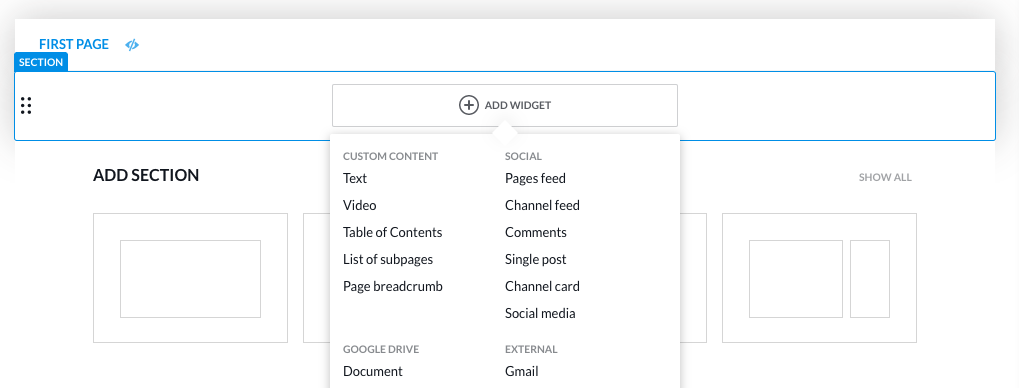
After adding the widget to the page, you should see "Developer error" -message.
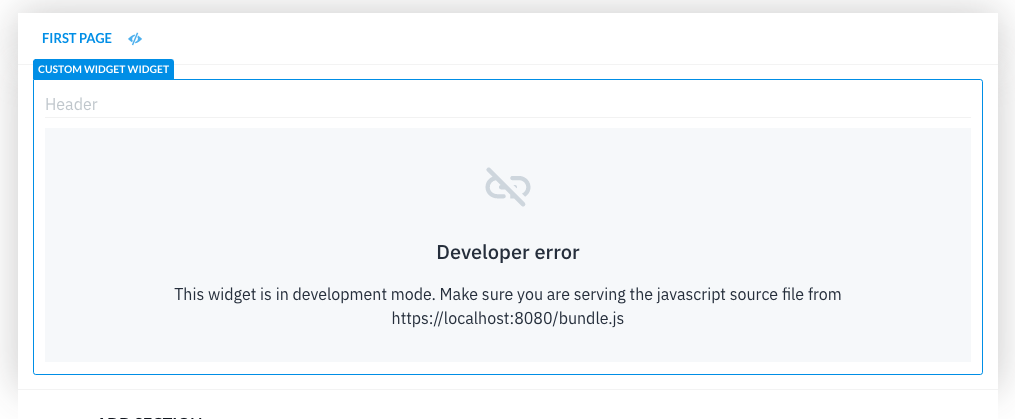
To get your widget to display here, we need the widget slug from the admin panel. Then we need to add it to the Accordion Widget example index.js -file you can get from here https://github.com/happeo/accordion-widget.
Paste your widget slug to the slug constant.
import React from "react";
import AccordionWidget from "./AccordionWidget";
class happeoCustomReactWidget extends HTMLElement {
connectedCallback() {
const uniqueId = this.getAttribute("uniqueId") || "";
const mode = this.getAttribute("mode") || "";
ReactDOM.render(
<AccordionWidget id={uniqueId} editMode={mode === "edit"} />,
this,
);
}
}
const slug = "slug-here"; // <-- Your slug here
window.customElements.get(slug) ||
window.customElements.define(slug, happeoCustomReactWidget);
After the slug is added, run npm i && npm start
. Then refresh your Happeo page with the custom widget added to it and ta'da, you are ready to go.
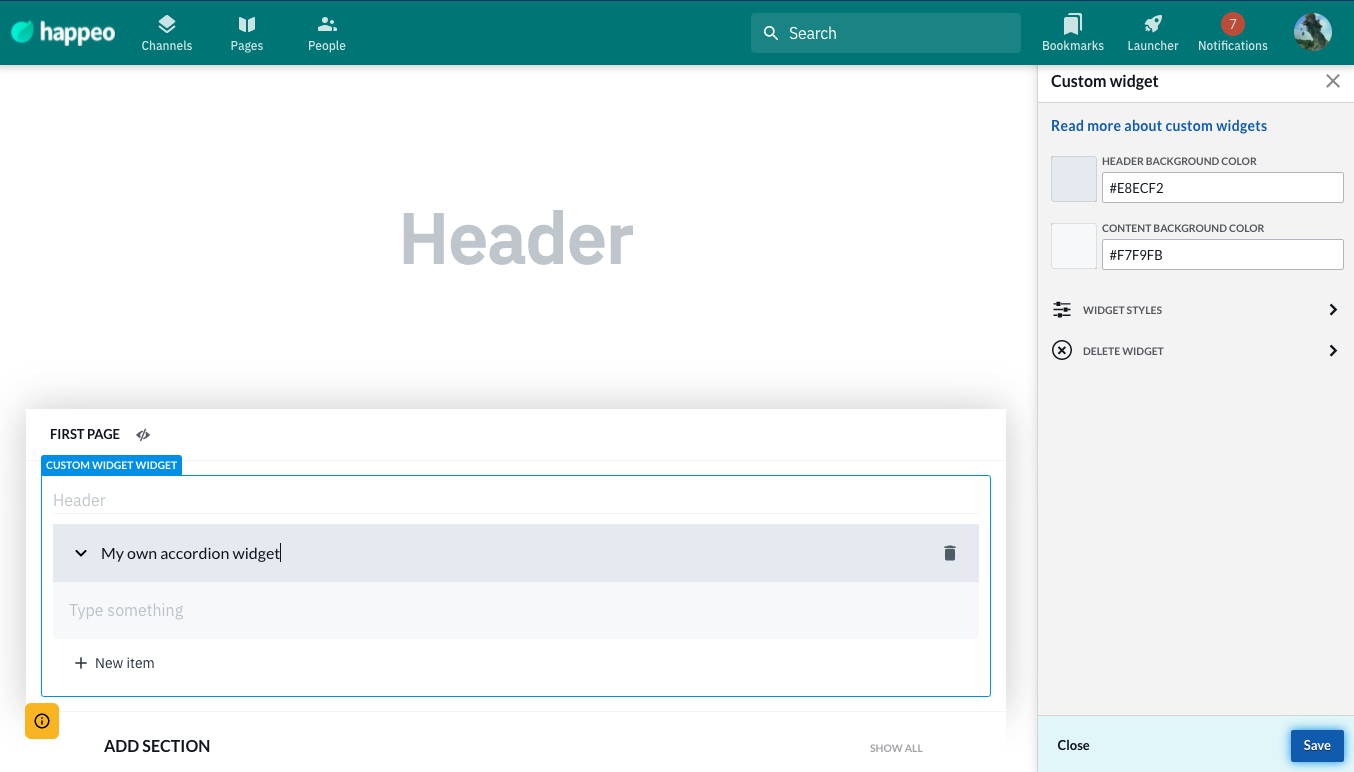
At this point we recommend examining the documentation in the custom widget templates (https://github.com/happeo/custom-widget-templates) and the WidgetSDK (https://github.com/happeo/widgets-sdk) repos as these go into detail about the properties and usage of the SDK.
TL;DR
- The widget element receives the following props:
uniqueId
,mode
&location
(more may be added later, check github repos if there are changes) - WidgetSDK is initiated by importing it and calling
const api = await widgetSDK.api.init(uniqueId);
where theuniqueId
is the one given in the element props.
Developing in Localhost
In case you do not have access to Happeo Pages for the time being. You can start developing in the localhost environment. Localhost development will use Mocked data in Widget-SDK API so you do not have access to Happeo services. We strongly recommend that you do development in Pages to get more end-user ui experience.
- git clone https://github.com/happeo/custom-widget-templates
- cd React && npm i
- npm run dev
- Open your browser to the localhost:8080 address.
Designing a good widget
In order to design a good widget, check the list of considerations to make from here.
Security
Please read the security considerations article:
https://developers.happeo.com/docs/security-and-quality-standards
Troubleshooting
If there's some wonky behaviour or you don't see your widget, check the following:
- Slug is correct (compare it what you can see in the DOM)
- The render method should show you something (edit mode vs view mode, no content, etc...)
- There are no conflicts in the used NPM libraries. The webpack utilises React, Shared Components and Happeouikit libraries directly from the app runtime, but version conflicts may cause havoc. Try removing the dependencies from the webpack and notify us through our support.
Updated about 2 years ago